There is a very nice way to navigate through the code using VS Code, just pressing Ctrl + Click
, it will take you to any definitions or declaration. This is achieved using one of the many plugins we can install. Since we are coding in C/C++ we must install the following extension.
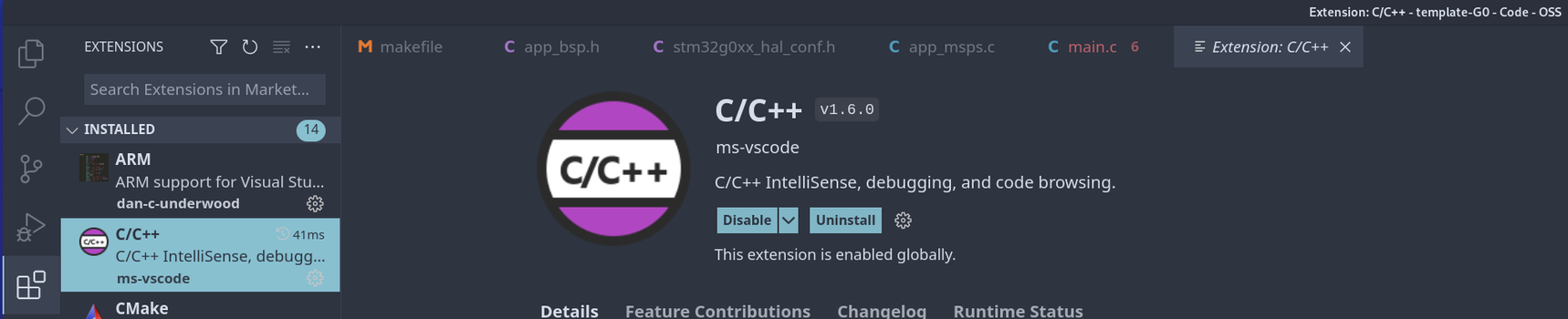
This is mainly a linter, that means it will try to tell you in advance if somethings is wrong with your code prior to be compile, but it is necessary to configure it the right way in order to get the best results. BUT remember it does not replace the fact that you have to compile the code and pay attention to compiler messages ( warnings and errors ).
Now we must create the following configuration file inside folder .vscode. In case you don’t have the folder .vscode in your project folder just type mkdir .vscode
and c_cpp_properties.json
$ code .vscode/c_cpp_properties.json
Now type the following inside. This is a json configuration file. If you are using the temeplate-g0 in linux you wont need to change anything
{
"configurations": [
{
"name": "ARM none eabi GCC",
"includePath": [
"${workspaceFolder}/app",
"${workspaceFolder}/cmsisg0/core",
"${workspaceFolder}/cmsisg0/registers",
"${workspaceFolder}/halg0/Inc",
"${workspaceFolder}/halg0/Inc/Legacy"
],
"defines": [
"STM32G0B1xx",
"USE_HAL_DRIVER"
],
"cStandard": "gnu11",
"compilerPath": "/usr/bin/arm-none-eabi-gcc",
"intelliSenseMode": "gcc-x64"
}
],
"version": 4
}
Settings
Time to explain each part of the file in case you need to add some extra paths or definitions
Where is says includePath you should write all your project paths within header files, only headers ( .h ) it is not necessary to indicate path with source files ( .c ). And only the paths, you do not need to write header files names.
"includePath": [
"${workspaceFolder}/app",
"${workspaceFolder}/cmsisg0/core",
"${workspaceFolder}/cmsisg0/registers",
"${workspaceFolder}/halg0/Inc",
"${workspaceFolder}/halg0/Inc/Legacy"
],
In case your projects uses global definitions you must write those definitions in section defines, remember the global defines are the ones written in the makefile
"defines": [
"STM32G0B1xx",
"USE_HAL_DRIVER"
],
Very important to indicate where in your computer is installed the ARM GCC compiler
"compilerPath": "/usr/bin/arm-none-eabi-gcc",
If you are using windows and git bash you have two options, to set this path windows style with double dashes
"compilerPath": "C:\\ProgramData\\chocolatey\\bin\\arm-none-eabi-gcc.exe",
or the same path but unix style
"compilerPath": "/c/ProgramData/chocolatey/bin/arm-none-eabi-gcc.exe",
So now with the Intellisense extension configured it is possible to Ctrl + click
on every symbol from your program and the file where is defined will open, and no only that but also if there is a problem with some symbol not defined the plugin will marked in red.