Timers can be configured in 2 modes.
Reload-mode: This mode allows the timer to execute again once that timer finishes.
One-shot: Execute timer only one time.
This example shows how work each mode, one button configured allows iterate between them. By default, Timer is configured in Reload-mode, but once that button is pressed the timer mode changes to one-shot, but if the button is pressed again Timer mode changes to default mode.
Code Example:
#include "bsp.h"
#define TIMER_ID (void *const)1 /* Timer ID */
TimerHandle_t xTimer; /* Handler of Timer */
static void TaskReadButton( void* parameter ); /* Task To Read Button */
static void vTimerCallback( TimerHandle_t pxTimer ); /* Function Callback of timer */
int main( void )
{
HAL_Init();
SEGGER_SYSVIEW_Conf();
SEGGER_SYSVIEW_Start();
xTimer = xTimerCreate( "Timer", 250, pdTRUE, TIMER_ID, vTimerCallback ); /* Creation of Timer */
xTimerStart( xTimer, 0 ); /* Start Timer */
xTaskCreate( TaskReadButton, "Task_Read_Button", 128u, NULL, 1u, NULL ); /* Register Task */
vTaskStartScheduler(); /* Execute Kernel */
}
/* Task To Read Button and change Reload mode of Timer */
static void TaskReadButton( void* parameter )
{
UNUSED(parameter);
uint32_t ReadPIN = 0; /* Variable to store Pin Button State */
for(;;)
{
ReadPIN = HAL_GPIO_ReadPin( GPIOB, GPIO_PIN_7 ); /* Check if button is pressed */
if( ReadPIN == GPIO_PIN_RESET ) /* If button was pressed Check Reload mode of Timer */
{
if( xTimerGetReloadMode(xTimer) == pdTRUE ) /* Check Reload mode of Timer */
{
vTimerSetReloadMode( xTimer, pdFALSE ); /* Change to one-shot mode */
SEGGER_SYSVIEW_PrintfHost("Change Reload Mode to pdFALSE");
}
else
{
vTimerSetReloadMode( xTimer, pdTRUE ); /* Change to Reload-mode */
xTimerStart(xTimer,0); /* Start Timer */
SEGGER_SYSVIEW_PrintfHost("Change Reload Mode to pdTRUE");
}
}
vTaskDelay( 40 / portTICK_PERIOD_MS ); /* Read button each 40ms */
}
}
/* Callback function of Timer to blink a led */
void vTimerCallback( TimerHandle_t pxTimer )
{
HAL_GPIO_TogglePin( GPIOC, GPIO_PIN_0 ); /* Blink a LED to know the Reload mode of timer */
SEGGER_SYSVIEW_PrintfHost("Timer Callback");
}
SystemView output:
The Terminal shows the message of a callback execution of the Timer register in Reload mode, notice that the callback is executed over and over every 250ms.
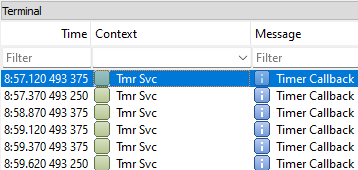
TimeLine:
When the application starts, the timer is registered by default with a period of 250 ticks and configured with the auto reload mode enabled. the timeline shows the execution of timer callback every 250 ticks. |
![]() ![]() |
When the button is pressed, the task detect the change and disable the reload mode of the timer, configuring it as a one-shot.
|
![]() |
Now the timer will execute the callback one more time after finishing the period of 250 ticks.
|
![]() ![]() |
Finally, the terminal shows that the button is pressed again and Callback is running concurrently. Check the elapsed time between messages, the button is pressed 2 seconds later.
| ![]() |