Code Example:
#include "bsp.h"
static void vTaskTemplate( void *pvParameters ); /* Declaration of Task function */
int main( void )
{
HAL_Init( );
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
xTaskCreate(vTaskTemplate, "task1", 240, (void*)"Tarea 1\n", 1, NULL); /* Register on Kernel the task1 with priority 1 */
xTaskCreate(vTaskTemplate, "task2", 240, (void*)"Tarea 2\n", 1, NULL); /* Register on Kernel the task2 with priority 1 */
xTaskCreate(vTaskTemplate, "task3", 240, (void*)"Tarea 3\n", 1, NULL); /* Register on Kernel the task3 with priority 1 */
vTaskStartScheduler(); /* Execute the Kernel */
return 0u;
}
static void vTaskTemplate( void *pvParameters )
{
char *pcTaskString = (char *)pvParameters; /* Obligatory cast to convert void parameter to char type */
for(;;)
{
SEGGER_RTT_printf( 0, pcTaskString ); /* Parameter message */
HAL_Delay(1000); /* Simple Delay of HAL library */
}
}
In this new program, we create three tasks from the same function. We use the fourth parameter of the xTaskCreate
function to pass a "parameter" to the created function. With this parameter, we can display different messages in each Task. But how is this possible?. Very simple. Using the xTaskCreate
function, the kernel reserves memory space in which it registers the "context" of each "task." In this program, three different contexts are reserved in the kernel even though the same function is used.
SystemView Output
Three tasks is executing printing each of them a different message, Terminal shows that 3 messages are sent at the same time and repeat after 1 second.
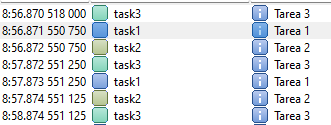
TimeLine:
Time line shows that task3 is executed first and after Task1 and task2, each task uses the same function and therefore has the same delay. The messages are sent at the same time. |
![]() |
When the delay time is over, the messages are sent again and repeat the process.
| ![]() |