Each task can be configured with a priority, which can be a value between 0 and configMAX_PRIORITIES - 1, A low priority number denotes a low-priority task where the IDLE task has a priority of zero.
configMAX_PRIORITIES
is defined on file “FreeRTOSConfig.h”Code Example:
#include "bsp.h"
static void vTask1( void *pvParameters ); /* Declaration of function Task1 */
static void vTask2( void *pvParameters ); /* Declaration of function Task2 */
int main( void )
{
HAL_Init( );
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
xTaskCreate(vTask1, "task1", 240, NULL, 2, NULL); /* Register on Kernel vTask1 with a priority of 2 */
xTaskCreate(vTask2, "task2", 240, NULL, 1, NULL); /* Register on Kernel vTask2 with a priority of 1 */
vTaskStartScheduler(); /* Execute the kernel */
return 0u;
}
static void vTask1( void *pvParameters )
{
for(;;)
{
SEGGER_RTT_printf(0, "Tarea 1 ejecutandose\n"); /* Friendly message 1 */
HAL_Delay(1000); /* Simple Delay */
}
}
static void vTask2( void *pvParameters )
{
for(;;)
{
SEGGER_RTT_printf(0, "Tarea 2 ejecutandose\n"); /* Friendly message 2 */
HAL_Delay(1000); /* Simple Delay */
}
}
The code is very similar to the first exercise, but here the two created tasks have different priorities. Observe the program's output and deduce the conclusion that can be reached. Modify lines 29 and 40 with the following function: vTaskDelay( 500 );
Observe the program's output again and deduce why it is different this time.
vTaskDelay
function refers to and represents. Speaking of priorities, what is the maximum priority I can assign to a task?SystemView Output:
Terminal shows that a Task with high priority executes and prints the message on the terminal, but this task never ends, printing on the terminal the same message without executing the second task.
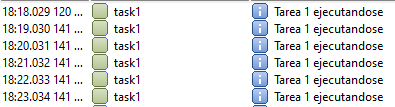
TimeLine:
If we observe the timeline, Task1 is always executing and never changes to Task2.
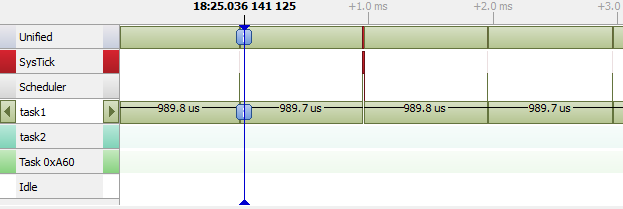
This happens because Task1 has the high priority and the task never executes a function to change the task state, Scheduler will execute the task with a ready state and the same priority. This means that when Task1 finished is in the “ready state”, the scheduler will execute again by the task state and priority.