The IDLE task is created automatically when the RTOS scheduler is started to ensure that there is always at least one task to execute. IDLE task is created with a lower priority of zero, to prevent that task don't consume CPU time when other task have a higher priority.
IDLE task hook is a function that is executed each cycle of the IDLE task. Allows to execute IDLE hook in the same priority of the Idle task without blocking the task.
Code Example:
#include "bsp.h"
#define RESET 0 /* Define a parameter to reset a value */
static uint32_t ulIdleCount = 0; /* Global variable to count the time in IDLE */
void vApplicationIdleHook( void ); /* Declaration of function to execute in IDLE task */
static void vTask( void *pvParameters ); /* Declaration of Task function */
int main( void )
{
HAL_Init( );
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
xTaskCreate(vTask, "task", 240, NULL, 1, NULL); /* Register a Task on Kernel with a priority of 1 */
vTaskStartScheduler(); /* Execute the Kernel */
}
static void vTask( void *pvParameters )
{
for(;;)
{
SEGGER_RTT_printf(0, "ulIdleCount = %d", ulIdleCount); /* Print on terminal the time of Kernel */
ulIdleCount = RESET; /* Restart the Kernel count */
vTaskDelay( 250/portTICK_PERIOD_MS ); /*Task blocked by 250ms*/
}
}
void vApplicationIdleHook( void ) /* Function is called when IDLE task is executed */
{
ulIdleCount++; /* Inclrement the global variable to count the time */
}
In the file FreeRTOSConfig.h, identify the following line and change its value from zero to one:
#define configUSE_IDLE_HOOK 1
In the previous code, we enabled the "idle hook" option, which allows us to insert our own application code into the kernel's IDLE task, or more precisely, into the function that the kernel calls when no task is available to enter the "Running" state. A simple increment of a global variable is executed within the idle function, and a straightforward task is responsible for displaying the variable's count on the screen every 250ms.
SystemView Output
The terminal shows the created task which prints on the terminal the current count of the global variable, when the value is printed the task will restart the global variable.
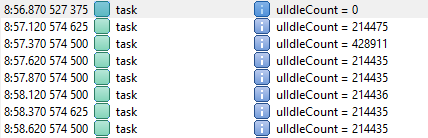
TimeLine:
The timeline shows the task execution when it prints the variable with a 0 value. After, in the timeline, the execution of the IDLE task is waiting for the Ready state of the task., each tick Idle task is executed.
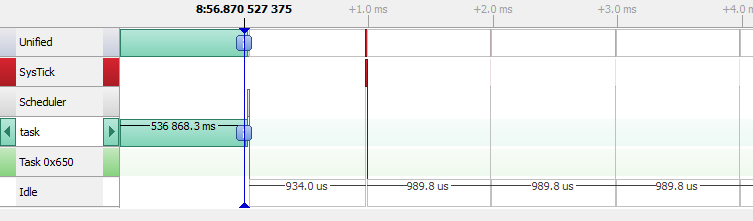