Similar to the last example, this application creates 2 tasks to blink 2 different LEDs. Each task is in charge of blink a specific LED.
Code Example:
#include "bsp.h"
/*Functions to act as tasks*/
static void vTask1( void *parameters );
static void vTask2( void *parameters );
int main( void )
{
HAL_Init( ); /*Inicializamos la librerÃa*/
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
/*register first task using the function vTask1 with (128*4) bytes de stack, no parameters
priority of 1 and no task handler registered*/
xTaskCreate( vTask1, "Task1", 128u, NULL, 1u, NULL );
/*register second task using the function vTask2 with (128*4) bytes de stack, no parameters
priority of 1 and no task handler registered*/
xTaskCreate( vTask2, "Task2", 128u, NULL, 1u, NULL );
/*run the RTOS scheduler*/
vTaskStartScheduler( );
return 0u;
}
/*Simple task that initialize pin C0 as output and toggle the pin state every 1000ms*/
static void vTask1( void *parameters )
{
GPIO_InitTypeDef GPIO_InitStruct; /* GPIO PIN Handler */
__HAL_RCC_GPIOC_CLK_ENABLE( ); /* Enable the clock for the port C */
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; /* Configure the Pin as output */
GPIO_InitStruct.Pull = GPIO_NOPULL; /* Configure pin as no pull up - no pull down */
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; /* Configure frequency of pin as High */
GPIO_InitStruct.Pin = GPIO_PIN_0; /* Configure PIN 0 */
HAL_GPIO_Init( GPIOC, &GPIO_InitStruct ); /* Initialize the GPIO */
for( ; ; )
{
HAL_GPIO_TogglePin( GPIOC, GPIO_PIN_0 ); /* change the state of pin 0, port c */
HAL_Delay( 1000u ); /* Delay of 1000ms */
}
}
/*Simple task that initialize pin C1 as output and toggle the pin state every 2000ms*/
static void vTask2( void *parameters )
{
GPIO_InitTypeDef GPIO_InitStruct; /* GPIO PIN Handler */
__HAL_RCC_GPIOC_CLK_ENABLE( ); /* Enable the clock for the port C */
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; /* Configure the Pin as output */
GPIO_InitStruct.Pull = GPIO_NOPULL; /* Configure pin as no pull up - no pull down */
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; /* Configure frequency of pin as High */
GPIO_InitStruct.Pin = GPIO_PIN_1; /* Configure PIN 1 */
HAL_GPIO_Init( GPIOC, &GPIO_InitStruct ); /* Initialize the GPIO */
for( ; ; )
{
HAL_GPIO_TogglePin( GPIOC, GPIO_PIN_1 ); /* change the state of pin 0, port c */
HAL_Delay( 2000u ); /* Delay of 2000ms */
}
}
SystemView Output
Notice that this timeline is similar to the last example, this time 2 tasks are running and the change of context made for the systick of the scheduler is more evident.
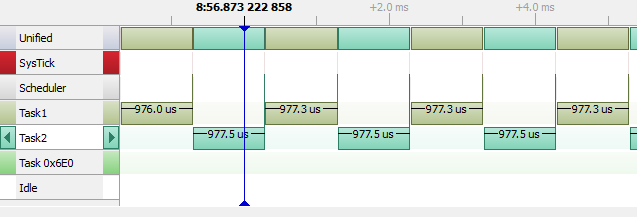
The normal execution of the tasks is not affected by the changes of context, Tasks going to blink the LEDs according to the Delay of each task.