The queue must be configured with a specific size, this must be calculated according to the data to manage in the application in a determined time before reading data from the Queue. For example, if Queue is set to receive less data that is sent, there will be data lost.
An example where Queue lost data:
#include "bsp.h"
#include <math.h>
#define NUMBER_OF_LEDS 8 /* Indicate the number of LEDs */
#define NONE_MESSAGES 0 /* Indicate that No messages available */
#define RESET_VALUE 0 /* Defualt value to restore variable */
static void WriteInQueue( void *parameters ); /* Task to select LED to be control */
static void ReadInQueue( void *parameters ); /* Task to control LED status */
QueueHandle_t Queue_LED;
int main( void )
{
HAL_Init( );
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
Queue_LED = xQueueCreate( 4, sizeof(uint16_t) ); /* Create the Queue with a buffer size of 4 elements of 16 bits */
xTaskCreate( WriteInQueue, "Task_Write_Queue", 128u, NULL, 1u, NULL ); /* Register a Task */
xTaskCreate( ReadInQueue, "Task_Read_Queue", 128u, NULL, 1u, NULL ); /* Register a Task */
vTaskStartScheduler( ); /* initilize the kernel */
return 0u;
}
static void WriteInQueue( void *parameters )
{
UNUSED( parameters );
/* Array of data to Send, 8 data must be send to Queue */
uint16_t Led_Pin[NUMBER_OF_LEDS] = { GPIO_PIN_0, GPIO_PIN_1, GPIO_PIN_2, GPIO_PIN_3, GPIO_PIN_4, GPIO_PIN_5, GPIO_PIN_6, GPIO_PIN_7 };
static uint16_t i = 0; /* Variable to iterate between array elements */
for( ;; )
{
xQueueSend( Queue_LED, &Led_Pin[i], 0 ); /* Send the current element of the Array to the Queue */
i++; /* Increment iteration count */
if (i >= NUMBER_OF_LEDS) /* Check if iteration of the 8 elements is done */
{
i = RESET_VALUE; /* Reset the Iteration Value to restart to init position of array */
}
vTaskDelay(200 / portTICK_PERIOD_MS); /* Send a element each 200ms, send all the array will cost 1600ms */
}
}
static void ReadInQueue( void *parameters )
{
UNUSED( parameters );
uint16_t dataRead; /* Variable to store data of Queue */
for( ;; )
{
while ( uxQueueMessagesWaiting( Queue_LED ) > NONE_MESSAGES )
{
xQueueReceive( Queue_LED, &dataRead, 0 ); /* Read the data of the Queue */
HAL_GPIO_TogglePin( GPIOC, dataRead ); /* Change the LED Status */
uint32_t res = log2(dataRead);
SEGGER_SYSVIEW_PrintfHost( "LED %d", res );
}
vTaskDelay(1600 / portTICK_PERIOD_MS); /* Read Queue buffer each 1600ms, when all the elements must be in Queue */
}
}
SystemView Output:
When the application is executed we can observe that Task ReadInQueue()
only receives from the Queue buffer 4 elements from the array Led_Pin[NUMBER_OF_LEDS]
in task WriteInQueue()
. The rest of the data is lost, this can happen if the Queue buffer fills.
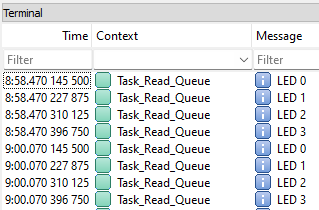
TimeLine:
Task WriteInQueue() finishes execution and the Queue buffer is full. The image shows the execution of task ReadInQueue() , all the buffer must be read and print only the messages received.
|
![]() |
If you compare the time of task execution with the time of this image, you will notice that the task is executed again after 1600ms ( The period of this task ).
|
![]() |