Let’s do something simple but interesting. In this example an interrupt trigger the Task Event to toggle a led.
#include "bsp.h"
#include "RTOS.h"
#define EVENT0 (1u << 0) // Bit 0 of the event bit mask
static OS_STACKPTR int TaskStack[128]; // Task Stack
static OS_TASK TaskCB; // Task control structure
static void Task(void);
int main( void )
{
OS_Init(); // Initialize embOS (must be first)
HAL_Init();
OS_InitHW(); // Initialize Hardware for embOS
OS_TASK_CREATE( &TaskCB, "DEMO TASK", 100, Task, TaskStack ); // Creating the task
OS_Start(); // Start multitasking
return 0u;
}
static void Task(void)
{
__HAL_RCC_GPIOC_CLK_ENABLE(); // Enable GPIOC Clock
__HAL_RCC_GPIOB_CLK_ENABLE(); // Enable GPIOB Clock
OS_TASKEVENT MyEvents; // This is our bit mask whith a 32 bits width
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Pin = GPIO_PIN_0;
HAL_GPIO_Init( GPIOC, &GPIO_InitStruct );
GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; // We are using de falling interrupt for the button
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Pin = GPIO_PIN_5;
HAL_GPIO_Init( GPIOB, &GPIO_InitStruct );
HAL_NVIC_SetPriority( EXTI4_15_IRQn, 2, 0); // Setting the Interrupt priority
HAL_NVIC_EnableIRQ( EXTI4_15_IRQn); // Enabling the IRQ
while(1)
{
MyEvents = OS_TASKEVENT_GetBlocked( EVENT0 ); // Wait for event bit 0
if(MyEvents & EVENT0 )
{ //* If the bit 0 is set, the EVENT0 is triggered and the pin 0 is toggle
SEGGER_SYSVIEW_PrintfHost( "EVENT 0" );
HAL_GPIO_TogglePin( GPIOC, GPIO_PIN_0 );
}
}
}
void HAL_GPIO_EXTI_Falling_Callback( uint16_t GPIO_Pin )
{ // Once the button is pressed the Falling callback function is called
OS_INT_Enter(); // Informing embOS that interrupt code is executing.
OS_TASKEVENT_Set(&TaskCB, EVENT0); // Triggering EVENT0
OS_INT_Leave(); // Informing embOS that the end of the interrupt routine has been reached
}
ints.c
void EXTI4_15_IRQHandler( void )
{
HAL_GPIO_EXTI_IRQHandler( GPIO_PIN_5 );
}
SYSTEMVIEW
Build and flash the program and then run systemview.

Notice how the task no longer runs at predefined time periods, instead it runs whenever the interrupt is triggered (by pressing a button in my case) and activates the task event.

Looking closer you can see how when the interrupt is triggered and the event is set, the “DEMO TASK” executes its routine. In the Event List you can see the behavior that I previously showed you on the timeline. This behavior is the same for the rest of the times I pressed the button.
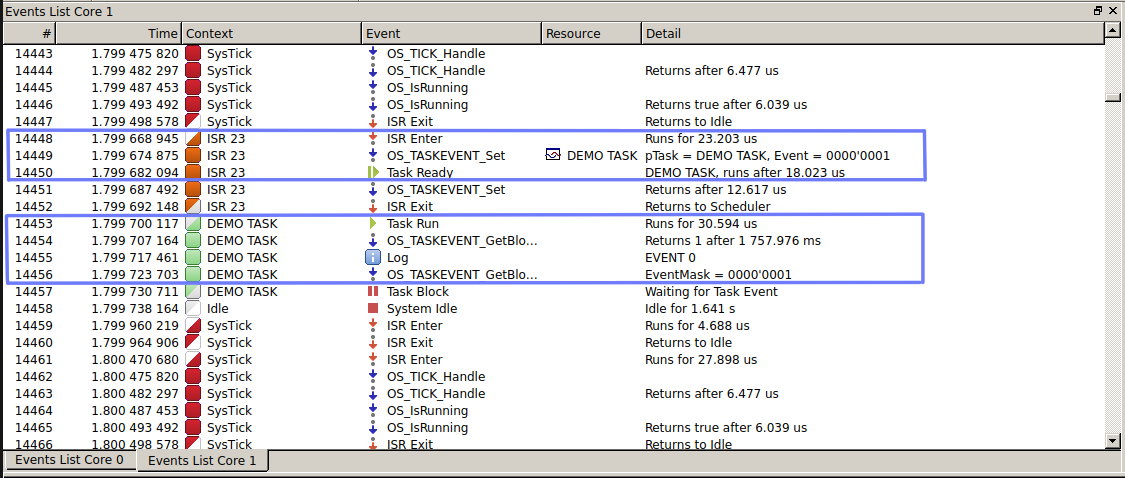