In this example a task event is triggered when a software timer reach its timeout. The main task is really simple we just have to create the Task where the event will be and the timer.
#include "bsp.h"
#include "RTOS.h"
#define EVENT0 (1u << 0) // Bit 0 of the event bit mask
static OS_STACKPTR int TaskStack[128]; // Task Stack
static OS_TASK TaskCB; // Task control structure
static OS_TIMER Timer; // Timer Control Structure
static void Task(void); // Task routine
static void Callback(void); // Timer callback
int main( void )
{
OS_Init(); // Initialize embOS (must be first)
HAL_Init();
OS_InitHW(); // Initialize Hardware for embOS
OS_TASK_CREATE( &TaskCB, "EVENT TASK", 100, Task, TaskStack ); // Creating the task.
OS_TIMER_CREATE(&Timer, Callback, 1000u); // Creating the software timer
OS_Start(); // Start multitasking
return 0u;
}
static void Task(void)
{
__HAL_RCC_GPIOC_CLK_ENABLE(); // Enable GPIOC Clock
OS_TASKEVENT MyEvents; // This is our bit mask whith a 32 bits width
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Pin = GPIO_PIN_0;
HAL_GPIO_Init( GPIOC, &GPIO_InitStruct );
while(1)
{
MyEvents = OS_TASKEVENT_GetBlocked( EVENT0 ); // Wait for event bit 0
if(MyEvents & EVENT0 )
{ // If the bit 0 is set, the EVENT0 is triggered and the pin 0 is toggle
HAL_GPIO_TogglePin( GPIOC, GPIO_PIN_0 );
}
}
}
static void Callback(void)
{
OS_TASKEVENT_Set( &TaskCB, EVENT0 ); // Triggering the EVENT0
OS_TIMER_Restart(&Timer); // Function to restart the timer
}
SystemView
Build and flash the program and then run systemview. First, let’s look at the timeline to see that every time the callback is executed the event task is also executed.

Looking closer we can see this behavior.

In the event list and terminal window you can check in more detail this program.
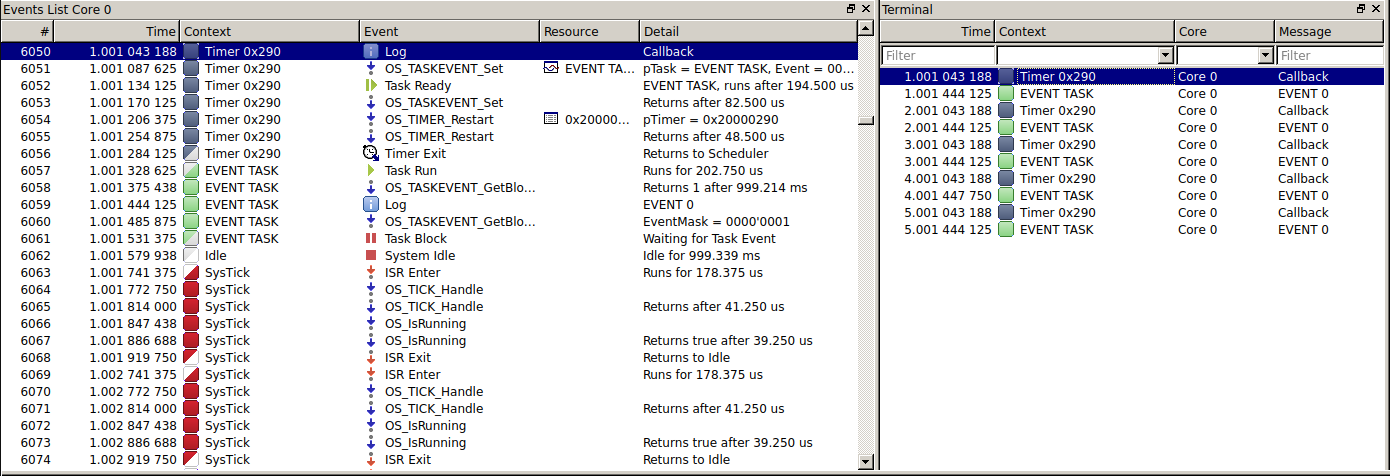