Code Example:
#include "bsp.h"
#define TIMERS_N 5 /* Number of timers to use */
TimerHandle_t xTimer[TIMERS_N]; /* Timer handler */
void vTimerCallback( TimerHandle_t pxTimer ); /* Funcion Callback */
int main( void )
{
unsigned long i; /* Variable to iter in For loop */
HAL_Init();
/*enable RTT and system view*/
SEGGER_SYSVIEW_Conf( );
SEGGER_SYSVIEW_Start( );
/* Creation of 5 Timers with ID from 1 to 5, using the same callback */
for( i = 1; i <= TIMERS_N; i++ )
{
xTimer[i-1] = xTimerCreate("timer", 150*i, pdTRUE, i, vTimerCallback); /* Create the 5 Timers with the same callback */
xTimerStart( xTimer[i-1], 0 ); /* Start the Timers */
}
vTaskStartScheduler(); /* Kernel execution */
}
void vTimerCallback( TimerHandle_t pxTimer )
{
int lTimerID = (int)pvTimerGetTimerID( pxTimer ); /* Get the timer ID */
SEGGER_RTT_printf( 0, "Tiempo cumplido de timer: %d", lTimerID ); /* Print a message on terminal of a Timer ID ends */
}
Through a for loop, 5 timers are created, and each handler is stored in an array. When creating each timer, different timeouts are assigned to each one, but a single callback function is used, shared among all timers.
Within the callback function, the function pvTimerGetTimerID
is used along with the parameter pxTimer
to extract the ID of the expired timer. This is used to display the correct message on the screen. However, there seems to be a discrepancy, as only 4 timers were created. Why is that?
SystemView Output:
The Terminal shows the callback function that prints a message with the ID of each timer that reaches the configured counter. Where each timer is configured with a different period.
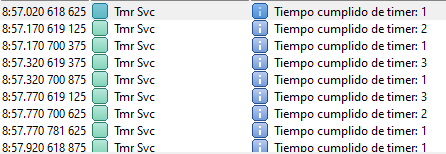
Timeline:
Image of the initialization of functions, where the timers are created and started.
|
![]() |
The period for Timer 1 is 150 ticks. Observe that the timer callback is executed exactly after the 150 ticks. |
![]() |
The period of Timer 2 is 300 ticks that can be observed on timeline that matches the execution of callback of both timers, Timer 2 and Timer 1. | ![]() |
The period of timer 3 is 450 ticks, observe that Times is exactly matched with the period and the callback function. This time callback of timer 3 and Timer 1 is executed. |
![]() |
The period of Timer 4 is 600 ticks, Compare the time on image with the image of the timers start. Timeline shows the callback execution of Timer 4, Timer 2 and Timer 1. |
![]() |
The period of Timer 5 is 750 ticks, Similarly to the past image, compare times between images. this time timeline shows the callback execution of timer 5, timer 2 and timer 1. | ![]() |